Python's Object Oriented Programming (OOPs) | Blog 1
About class, object, reference variables, self variable, constructors and Instance Varaiable
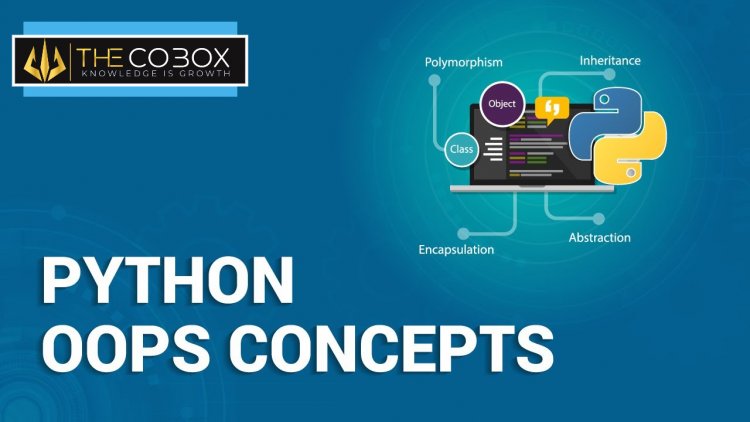
What is Class:
- In Python everything's an object. To create objects we required some Model or Plan or Blueprint, which is nothing but class.
- We can write a class to represent properties (attributes) and actions (behavior) of object.
- Properties can be represented by variables
- Actions can be represented by Methods.
- Hence class contains both variables and methods.
How to Define a class?
We can define a class by using the class keyword.
Syntax:
class className:
''' documentation string '''
variables:instance variables,static and local variables
methods: instance methods,static methods,class methods
Documentation string represents the description of the class. Within the class doc string is always optional. We can get doc strings by using the following 2 ways.
- print(classname.__doc__)
- help(classname)
Example:
class Student: |
Within the Python class we can represent data by using variables.
There are 3 types of variables allowed.
- Instance Variables (Object Level Variables)
- Static Variables (Class Level Variables)
- Local variables (Method Level Variables)
Within the Python class, we can represent operations by using methods. The following are various types of allowed methods
- Instance Methods
- Class Methods
- Static Methods
Example for class:
class Student: |
What is Object:
Physical existence of a class is nothing but an object. We can create any number of objects for a class. Syntax to create object: referencevariable = classname()
Example: s = Student()
What is Reference Variable:
The variable which can be used to refer to an object is called a reference variable. By using reference variables, we can access properties and methods of objects.
Program: Write a Python program to create a Student class and create an object to it. Call the method talk() to display student details
class Student: |
Output:
D:\thecobox>py test.py
Hello My Name is: Durga
My Rollno is: 101
My Marks are: 80
Self variable:
self is the default variable which is always pointing to the current object (like this keyword in Java) . By using self we can access instance variables and instance methods of objects.
Note:
- self should be first parameter inside constructor
def __init__(self):
- self should be first parameter inside instance methods
def talk(self):
Constructor Concept:
- Constructor is a special method in python.
- The name of the constructor should be __init__(self)
- Constructor will be executed automatically at the time of object creation.
- The main purpose of the constructor is to declare and initialize instance variables.
- Per object constructor will be executed only once.
- Constructor can take at least one argument(at least self)
- Constructor is optional and if we are not providing any constructor then python will provide default constructor.
Example:
def __init__(self,name,rollno,marks):
self.name=name
self.rollno=rollno
self.marks=marks
Program to demonstrate constructor will execute only once per object:
class Test: |
Output
Constructor execution...
Constructor execution...
Constructor execution...
Method execution...
Program:
class Student: |
Output
Student Name:Durga
Rollno:101
Marks:80
Student Name:Sunny
Rollno:102
Marks:100
Differences between Methods and Constructors:
Method |
Constructor |
1. Name of the method can be any name |
1. Constructor name should be always __init__ |
2. Method will be executed if we call that method |
2. Constructor will be executed automatically at the time of object creation. |
3. Per object, method can be called any number of times. |
3. Per object, Constructor will be executed only once |
4. Inside the method we can write business logic |
4. Inside Constructor we have to declare and initialize instance variables |
Types of Variables:
Inside Python class 3 types of variables are allowed.
- Instance Variables (Object Level Variables)
- Static Variables (Class Level Variables)
- Local variables (Method Level Variables)
- Instance Variables:
If the value of a variable is varied from object to object, then such type of variables are called instance variables.
For every object a separate copy of instance variables will be created.
Where we can declare Instance variables:
- Inside Constructor by using self variable
- Inside Instance Method by using self variable
- Outside of the class by using object reference variable
- Inside Constructor by using self variable:
We can declare instance variables inside a constructor by using self keyword. Once we create an object, automatically these variables will be added to the object.
Example:
class Employee: |
Output: {'eno': 100, 'ename': 'Durga', 'esal': 10000}
- Inside Instance Method by using self variable:
We can also declare instance variables inside the instance method by using self variables. If any instance variable is declared inside the instance method, that instance variable will be added once we call that method.
Example:
class Test: |
Output
{'a': 10, 'b': 20, 'c': 30}
- Outside of the class by using object reference variables:
We can also add instance variables outside of a class to a particular object.
class Test: |
Output {'a': 10, 'b': 20, 'c': 30, 'd': 40}
How to access Instance variables:
We can access instance variables within the class by using self variables and outside of the class by using object reference.
class Test: |
Output
10
20
10 20
How to delete instance variables from the object: 1. Within a class we can delete instance variable as follows
del self.variableName
- From outside of class we can delete instance variables as follows
del objectreference.variableName
Example:
class Test: |
Output
{'a': 10, 'b': 20, 'c': 30, 'd': 40}
{'a': 10, 'b': 20, 'c': 30}
{'a': 10, 'b': 20}
Note: The instance variables which are deleted from one object,will not be deleted from other objects.
Example:
class Test: |
Output
{'b': 20, 'c': 30, 'd': 40}
{'a': 10, 'b': 20, 'c': 30, 'd': 40}
If we change the values of instance variables of one object then those changes won't be reflected in the remaining objects, because for every object we separate copies of instance variables are available.
Example:
class Test: |
Output
t1: 888 999
t2: 10 20
What's Your Reaction?




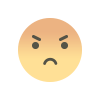

