4 || Identifiers, Keywords, Data types || Python Basic Full Course
This Lecture contain types of Identifies, List of keywords and List of data types with definitions of each type. Int value detail description.
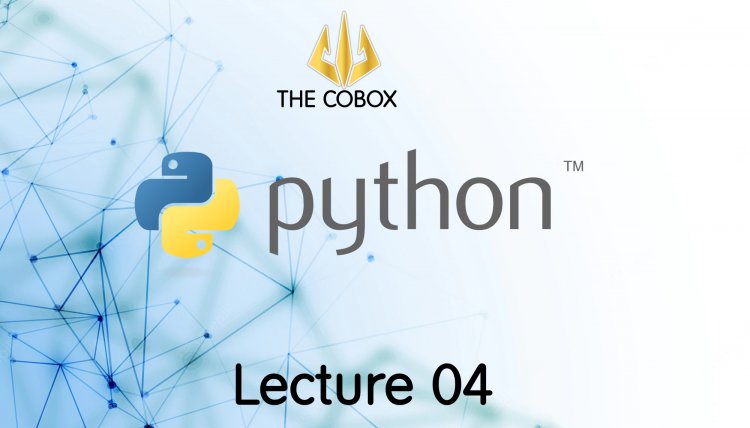
The identifier in Python:
The variable names, Method names, and Class names.
Rules to set identifiers:
- a to z , A to Z , 0-9, _
- Starts with digit
- Case sensitive
- Reserved words as identifiers
- No length limit
Special Cases:
- _x ===> private
- __x ===> strongly private
- __name__
- __add__
Reserved Keywords in Python :
There are 33 reserved words in python to represent some functionality are :
- True, False, None
- and, or, not, is
- if, else, elif
- while, for, continue, return, in, yield
- try, except, finally, raise, assert
- import, from, as, class, def, pass, global, nonlocal, lambda, del, with
Note :
- All reserved contains only symbols
- Except first 3 using the capital symbol
Data Types In Python:
14 types of datatypes in python are listed below:
- int
- float
- complex
- bool
- str
- bytes
- bytearray
- range
- list
- tuple
- set
- frozenset
- dict
- none
int : Contains only interger values like 10,20,100
float: contains only deicamal values like 1.25, 2.4
complex : contain complex number which have number in additin with complex value followed by (j)
bool : contains only two value true and false
str : If you want to represent String then we can use str
bytes : It returns a group of values which is non mutable
bytearray : It returns a group of values which is mutable
range : it contains range like 1 to 10, 2 to 500 and so on.
list : List of values Mutable
tuple : list of value but immutable
set : A set is an unordered and unindexed collection of unique elements. Mutable in nature
frozenset : same as set but immutable in nature.
dict : A set of Key value pairs in braces.
none : A none keyword is used to define null value.
1) int datatype :
Properties : int data type can only store interget values.
example: 10, 20, 55, 89 etc.
Code :
a = 10
type(a)
Output:
Types of interger values:
-
- Decimal values
- Type : Decimal (Base-10)
- Range : 0 to 9
- Example : a = 7878
- Binary values
- Type : Binary (Base-2)
- Range : 0 to 1
- Example : a =0B 1111 or a =0b 1111
- Octal form values
- Type : Octal (Base-8)
- Range : 0 to 7
- Example : a = 0o777
- Hexa decimal values
- Type : Hexa decimal (Base - 16)
- Range : 0-9, a-f OR 0-9, A-F
- Example : 0x or 0X
- Decimal values
What's Your Reaction?




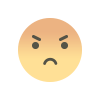

